The history of every major Galactic Civilisation tends to pass through three distinct and recognisable phases, those of Survival, Inquiry and Sophistication, otherwise known as the How, Why and Where phases. For instance, the first phase is characterised by the question ``How can we eat?'' the second by the question ``Why do we eat?'' and the third by the question ``Where shall we have lunch?''
So far we've seen actors (objects), roles the actors play (classes), scenes the actors play out (methods), a combination stage manager and producer (the Java interpreter), a combination playwright and director (the Java programmer), and an audience (the program's users). Just as every major theater has a backstage, however, with a whole warren of rooms full of lights, costumes, food, and other support services, to write Java programs we will also need props for the actors to handle (variables), stage directions the actors follow (statements), names for the actors (reference values), names for the characters that the actors will play (reference variables), and cues the actors give to each other (messages).
Theater | Java |
---|---|
actors | objects |
roles | classes |
scenes | methods |
playwright and director | programmer |
stage manager and producer | Java interpreter |
audience | program users |
 
|
|
props | variables |
stage directions | statements |
actor names | reference values |
character names | reference variables |
cues | messages |
The Actors' Roles---Java Classes
Let's write our first role, SimpleRole
;
by it's name it's clear that we're not competing with
Hamlet
, TheCherryOrchard
,
or TheImportanceOfBeingEarnest
.
To name a Java class it's usual to describe it (briefly),
capitalize all the words, then run them together;
this style is CamelCase.
The Java interpreter forces us to remove all spaces and punctuation
to make its job easier,
but we also want to remember what we meant when we first wrote the role.
Once we name a class, we can start specifying the behavior of the objects that will belong to the class by listing a sequence of statements, or requests to the Java interpreter, all inside braces. By the end of this chapter we'll have made a very small role describing an exceedingly nondescript actor whose brief time on stage will be spent dividing two small numbers and reporting the result. It'll be Waiting for Godot minus Vladimir, Lucky, Pozzo, and the boy---oh, and minus the withered tree, the stone, the country road, the sky, and Estragon's boots and hat; we will, however, keep Vladimir's turnips. As brutally simple---and boring---as our minuscule playlet will be, we'll start even smaller and grow our way there, picking up pieces of Java as we go. Here then is our first role:
class SimpleRole
{
}
Not too impressive, true. Right now the role is empty, but it's legal Java; it will work just fine. Of course, it also won't do anything since we haven't added any statements yet.
State and Behavior
Props are things that actors fiddle with while on stage; they can be in various states but they can't behave---that's what actors are for. Without props, the stage gets a bit barren (a Beckett play, perhaps), but props alone are boring because, usually, they just sit there. A gun in a Chekhov play can be cocked or uncocked, and it can be loaded or unloaded, but it won't hop from one state to the other by itself; it simply sits on the mantlepiece until one of the actors shoots another actor with it in Act III, Scene II.
Props usually don't behave on their own---they're picked up, put down, stolen, mislaid, eaten, or slept on by the actors. Similarly, variables in a Java program, like most props in a play, only have state, but no behavior---they continue in one mode until an actor changes their mode; they can't act on their own. Actors, on the other hand, can have both state and behavior. They may be hungry or full, asleep or awake, sitting or standing---that's part of their state; they may also eat, fall down, smoke, yawn, snooze, sneeze, and sing---that's part of their behavior. For an actor, "behaving" means "changing state".
Sometimes we'll ask an actor simply to sit still and just hold some props for other actors. Such an actor might just as well be a prop. Most actors though prefer to move about, talk to other actors, get hit on the head, drink, gamble, sleep with other actors, and, of course, have sword fights. They embody the whole life of the play. They're what the play is all about.
When we're designing a program, everything revolves around roles. Roles determine what functions we will be supporting, how they relate to each other, and how they collaborate with other roles. When we're building the program, though, everything revolves around classes. They specify what variables the objects will possess; what names, types, and values those variables will have; how the objects will manipulate their variables; what messages the objects can send to each other; and what the objects can do when they get a message. Finally, when we're running the program, everything revolves around objects. Variables help them remember their state, references help them interact with each other, and classes describe their state and behavior. They're what the program is all about.
The Actors' Props---Java Variables
Variables are the props we use in our plays. A variable is like a small box an actor carries around. Each of these boxes has a name, a value, and a type. Its name identifies it, its value is what's inside it, and its type specifies what values we can put into it. All it can do is hold values, it always has some value, it can only have one value at a time, and it can only hold values of its specific type. The particular set of values it will accept specifies its type, and the value presently in it specifies its state.
There are only four different types of props we need to know,
and we're going to look at three of them right now.
First, boolean
variables can hold one of only two values:
true
or false
.
Second, int
variables
can hold a whole number (or integer),
but only one within a certain range.
Third, double
variables can hold a decimal,
a number with fractional parts like 3.14159,
but only one within a certain range and to a certain precision.
We can keep any one of the above three types of values
in a variable of that type.
There is one more type of value that we can put in a fourth kind of variable,
and that is actor names,
but we won't see them for a while.
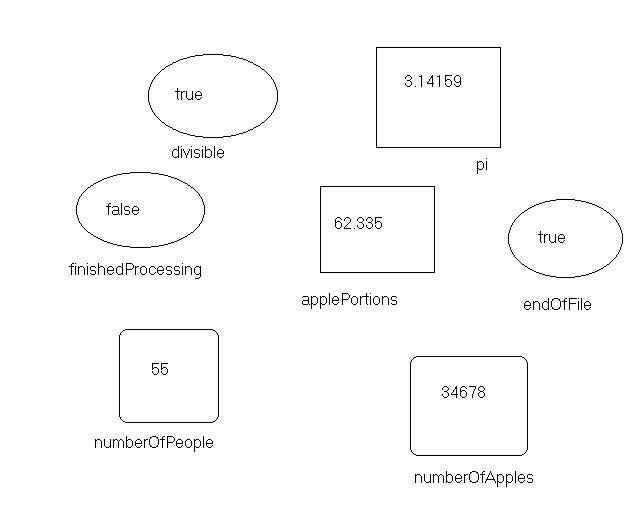
The Actor's Stage Directions---Java Statements
class SimpleRole
{
int numberOfTurnips;
double turnipsPerPerson;
boolean finishedDividing;
}
This is another legal Java class.
First, all statements must end with a semicolon.
Second, variable names, like class names, are in CamelCase,
except that they start with a lower-case letter.
The Java interpreter cares about letter case,
so SimpleRole, Simplerole, simpleRole
,
and simplerole
are four different names.
The Java interpreter, however, doesn't care about indentation,
but your human readers do,
so as a matter of style
it's common to indent all statements inside the class definition.
Our class now declares three props:
one int
variable,
one double
variable,
and one boolean
variable.
These three declaration statements
ask the stage manager to give any actor playing this role
its own copies of the above three props.
So far, though, the role doesn't specify anything for such actors to do
using their copies of those three props.
The Actor's Scenes---Java Methods
class SimpleRole
{
int numberOfTurnips;
double turnipsPerPerson;
boolean finishedDividing;
double divideTurnipsAmongPeople()
{
int numberOfPeople;
numberOfTurnips = 6;
numberOfPeople = 2;
turnipsPerPerson = numberOfTurnips / numberOfPeople;
finishedDividing = true;
return turnipsPerPerson;
}
}
We've now defined a method---an action,
defined by a named sequence of statements---that
all actors playing SimpleRole
will know how to perform;
it is a scene they can now play.
When cued to do so, an object enters some particular method
defined for its class
and executes the method
(does the actions that the statements within the method specify)
then exits, or "returns from", the method
when it executes a return
statement.
Every statement, aside from any declaration statements,
is an executable statement.
This method,
like most things that we must describe to the Java interpreter,
must have a name and a type.
First, its name is divideTurnipsAmongPeople
,
which looks just like a variable name.
To distinguish method names from variable names,
method names will appear with trailing parenthese, like this:
divideTurnipsAmongPeople()
.
Second, its type is double
,
just like the variable turnipsPerPerson
.
There is a difference though;
a variable's type specifies what values it can accept,
but a method's type specifies what values it can produce.
Here, the type of the value that an actor returns when exiting the method
will be double
.
Thus, all the values an actor can produce after executing this method
will each belong to the set of double
values.
So we can put any of those values into a double
variable.
Using Assignments and Operators
To understand divideTurnipsAmongPeople()
we also need to know that we can copy
the contents of one variable x
to another variable y
by saying:
y = x;
This is an assignment statement;
it asks the stage manager (the Java interpreter)
to copy the value that's inside the box labelled with the name
on the right-hand side of the statement
then put that copy into the box labelled with the name on the left-hand side.
The effect is to copy (or assign)
the value currently in variable x
(that is, the value in the box named x
)
to the variable y
(the value in the box named y
).
This wipes out whatever value was previously in y
but leaves x
's value intact.
(It is a sadness that, for historical reasons, Java uses ``=
''
for assignment, when a much less misleading symbol like
``<-
'' would be better; but the weight of history is heavy.)
Besides copying the values of variables into other variables, we can also put values into variables directly, which is the effect of an assignment statement like:
numberOfTurnips = 6;
This puts the value 6, which is of type int
since it's a whole number and it lies well within the range
of int
s,
into the variable named numberOfTurnips
,
whose type is int
.
We can also use various operators to compute new values given two or more variables (or operands) and put the result in yet another variable, which is the effect of the statement:
turnipsPerPerson = numberOfTurnips / numberOfPeople;
This statement asks the stage manager to divide
the value in the int
variable numberOfTurnips
by the value in the int
variable numberOfPeople
and put the result in the double
variable
turnipsPerPerson
.
An operator is like a tiny method;
thus ``/
'' is the same as a method called,
say, divide()
that accepts two numerical variables
and produces the result of dividing the value of one by the value of the other.
Even assignments are like tiny methods
(that is, if variables were like objects,
the variable on the left-hand side of an assignment statement
responds to ``=
'' as its cue and stores the value of the
right-hand side as its new value).
No matter what's on the right-hand side, though, the left-hand side of every assignment statement must be the name of a variable (that is, a box) since the Java interpreter must have somewhere to put the result of the right-hand side.
Creating an Actor
Although SimpleRole
now specifies some state (held in variables)
and behavior (described by methods),
it still does nothing.
It looks like it's storing the value 6
in the int
variable numberOfTurnips
,
the value 2 in the int
variable numberOfPeople
,
dividing the two of them,
storing the result
in the double
variable turnipsPerPerson
,
then setting the boolean
variable finishedDividing
to true
.
Then it's returning the result of the division to somebody.
In fact, though, nothing at all happens
because we don't yet have an actor to ask to do anything.
So let's create an actor to play SimpleRole
.
This isn't easy, however,
because at the beginning of the play there are no actors at all,
so we don't yet have an object that we can ask to create another object.
We get around this by adding a specially named method
that the stage manager can respond to.
In it we ask the stage manager to create an actor for us
and so start the play.
class SimpleRole
{
int numberOfTurnips;
double turnipsPerPerson;
boolean finishedDividing;
double divideTurnipsAmongPeople()
{
int numberOfPeople;
numberOfTurnips = 6;
numberOfPeople = 2;
turnipsPerPerson = numberOfTurnips / numberOfPeople;
finishedDividing = true;
return turnipsPerPerson;
}
public static void main(String[] parameters)
{
SimpleRole estragon;
estragon = new SimpleRole();
}
}
The strangely declared main()
method
is special because it tells the stage manager where the play begins;
it's like Act I, Scene I of a script.
The stage manager will automatically execute the main()
method
of any role if we declare it exactly as above.
Although the mysterious main()
method is short,
a lot has happened backstage on this last step,
not all of which is important just this minute.
For now, it's enough to know that inside the new method
we ask the stage manager
to create an actor using the ``new
'' operator.
Like any operator, we can think of new
as a tiny method;
in this case, it's short for
createAnActorThatWillPlayTheFollowingRole
,
followed by the name of the role we want the newly created actor to play,
followed by brackets.
(We won't find out why the brackets are necessary for several chapters.)
When the stage manager sees a new
operator,
it creates an actor that will play the role we name,
and creates copies of each of the props the role specifies for any such actor.
The new actor will then have props (variables) to remember its state
and scenes (methods) to determine its behavior.
Naming the Actor
While creating an actor, we must also name it if we're going to refer to it in future, which is the purpose of the left-hand side of the assignment statement:
estragon = new SimpleRole();
Here we're calling the new actor estragon
.
Or, more precisely, we're telling the stage manager
that we'll be referring to the newly created actor,
who will be playing SimpleRole
for the rest of its life,
by the reference variable estragon
.
Or, even more precisely, we're asking the stage manager
to put a copy of the real name of the new actor
(which we're simultaneously asking the stage manager to create)
into the reference variable named estragon
.
In theater terms, there's a difference between
the name of the character that an actor plays---in
this case, the character's name is estragon
---and
that actor's real name.
We, the playwright/directors, don't know---and could care less about---any
actor's real name;
all we need is the name of the character that actor plays.
When we say, for instance, "Romeo
kisses Juliet
",
we don't have to care that the real name of the actor
playing the Romeo
part is Leo DeCaprio.
Later on we'll see that we needn't even give an actor a character name.
Like extras in a crowd scene, actors can also play nameless roles.
Finally, just as with all the other variables declared earlier
we must declare the name and type of the new variable (estragon
)
to the Java interpreter.
We do that as follows:
SimpleRole estragon;
This declaration tells the Java interpreter
that the new variable is a reference variable
(since it will contain a reference value,
that is a reference to an object),
and that its type is SimpleRole
.
The Actors' Names---Java References
Reference values point to, or name, objects. Although they point to objects and are kept in variables, they are neither objects nor variables. We need them because objects need names. We can't have the characters that the actors play running around calling each other ``Hey, you'' all the time; that would make the play too confusing. And, just as in a play, each character can have many names, or aliases.
It may seem strange to obsess about names, but naming things, and using those names properly, is a big part of programming. It's especially important in Java programs where much of the action often consists of actors cuing each other by sending messages back and forth. Messages can't be sent unless names are first known.
We put reference values in reference variables,
just as we put boolean
values
in boolean
variables,
int
values in int
variables,
double
values in double
variables.
As with the three other types of variables,
reference variables are little boxes with names, types, and values,
except that the values in these particular boxes are reference values
(that is, actors' real names).
Unlike boolean
or int
or double
values,
the set of reference values that we can put in a reference variable
is theoretically unlimited---we could have any number of actors,
and any number of names to call them.
There is a big difference between the names in reference variables and the names of reference variables. The names they store are the names of actors (that is, names of objects), the names they have are the names of characters those actors play. It's the difference between what's inside a box (an actor's real name) and a label on the outside of the box (the name of the character that actor plays).
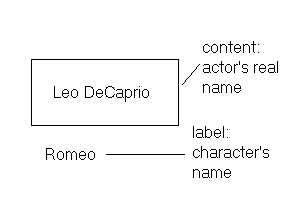
Further, and again just like the other three kinds of variables, every reference variable has a type. That type might be the same as the type of the object that the reference variable names, or it might be different. We'll explore that later on. For now, just remember that everything in Java must have a type.
The Actors---Java Objects
Objects are the players that strut and fret on our little stage. They can not only keep values as the four types of variables do, they can also modify those values---and they can ask other objects to modify their own values, too. Thus, objects can have state and behavior.
A variable, whether reference or otherwise, only has state:
all it can do is lay around and remember
what value it is currently storing
out of all the possible values it could be storing.
A boolean
variable, say,
would at any time either be holding the value true
or the value
false
.
Which one of the two it's presently holding determines its current state,
and that's all.
The same goes for int
variables, double
variables,
and reference variables.
An object, though, can have many variables,
so it can have much more complicated states,
and, on top of that, it can also behave:
it knows how to do things.
Part of an object's behavior might be to talk to itself or to other objects. It asks objects to do various things by sending messages, the theater equivalent of speaking a line of the script to another actor (or to itself). Receiving a message might cause those objects to change state and send other messages to yet more objects, one of which might be the original sender. Receiving a reply message might cause the original sender to change state and send out yet more messages. Thus, receivers can become transmitters---and transmitters receivers---perhaps together generating a vast and complex swarm of messages leading to massive state changes in numerous objects. Much of what happens in a well-designed Java program happens through objects sending messages back and forth.
The Actor's Cues---Java Messages
At last it looks like we finally have a working Java program. Alas, our program still does almost nothing. To recap, here's what we've done so far:
This isn't quite enough, however. Although the object that the reference variableWe've asked the stage manager to create a new actor that understands how to play
SimpleRole
and to reference it for us with (that is, use as its character's name) variableestragon
in Act I, Scene I (themain()
method).
We've given any
SimpleRole
actor some behavior (dividing two values and storing some values) by specifying thedivideTurnipsAmongPeople()
method.
We've given any
SimpleRole
actor some props to play with by declaring them within the class.
estragon
points to exists,
and has some props, and knows how to divideTurnipsAmongPeople()
,
we haven't sent it a message asking it to
divideTurnipsAmongPeople()
.
It knows how to behave,
but we haven't asked it to behave.
So let's do that next:
class SimpleRole
{
int numberOfTurnips;
double turnipsPerPerson;
boolean finishedDividing;
double divideTurnipsAmongPeople()
{
int numberOfPeople;
numberOfTurnips = 6;
numberOfPeople = 2;
turnipsPerPerson = numberOfTurnips / numberOfPeople;
finishedDividing = true;
return turnipsPerPerson;
}
public static void main(String[] parameters)
{
SimpleRole estragon;
estragon = new SimpleRole();
double portionsPerPerson;
portionsPerPerson = estragon.divideTurnipsAmongPeople();
}
}
We can ask any actor to execute any of its methods with the dot operator, as in the statement:
[actor's name].[actor's method's name];
This statement sends the actor the message:
"please execute your such-and-so method."
As with any other operator, the dot operator is again a tiny method.
With it, we can ask any actor to send such a message to any other actor,
or even to itself,
or, as in the main()
method of SimpleRole
,
we can ask the stage manager to send a message to an actor.
We just have to make sure that the receiving actor understands the message,
that is, that the receiving actor has a method by that name.
In this particular case,
the actor's real name (which is a reference value)
is hidden inside the reference variable named estragon
,
which is the name of the character the actor plays,
and we are asking that actor to execute
its divideTurnipsAmongPeople()
method.
So the message from the stage manager to estragon
is:
estragon.divideTurnipsAmongPeople();
An actor executes its methods only when asked to by another actor
(or by itself, or by the stage manager).
There is no such thing as an actorless action in Java.
In this most recent version of our role,
after creating and naming an actor,
we cue the actor by sending it a message asking it to execute
its divideTurnipsAmongPeople()
scene
(which, remember, is a method, not a variable,
even though it has a type---double
, in this case).
The actor, playing its divideTurnipsAmongPeople()
scene,
divides the number of turnips by the number of people,
saves the result, assigns a value to a boolean
variable,
then returns the portions per person.
Then we ask the stage manager to save
a copy of that double
value in a new double
variable,
portionsPerPerson
.
Finally, at long last, we now know that six divided by two yields three.
All right, we've created our first program, and it actually does something but it's severely limited in many ways---for example, it will only ever divide exactly the same two numbers, not to mention that when it runs nothing appears on our screen. Our next task is to fix those problems.